EENG 383
Lab 2 - Morse Code
InLab 2
Some self-guided
activities.
Lab 2 theory
During this weeks lab you will create a program that outputs a
sequence of illumination pulses that transmits a Morse code coded
message upper button is pressed.
Morse code is a method for transmitting text-based messages using a
series of short and long signals called dot and dash respectively.
The signal, in our case, will
be an LED that illuminates for a short or long period. The pulses
of LED illumination are separated by non-illuminated periods of variable
length. Each letter and number has a specific, predefined, pattern of
dots and dashes given in the figure below.
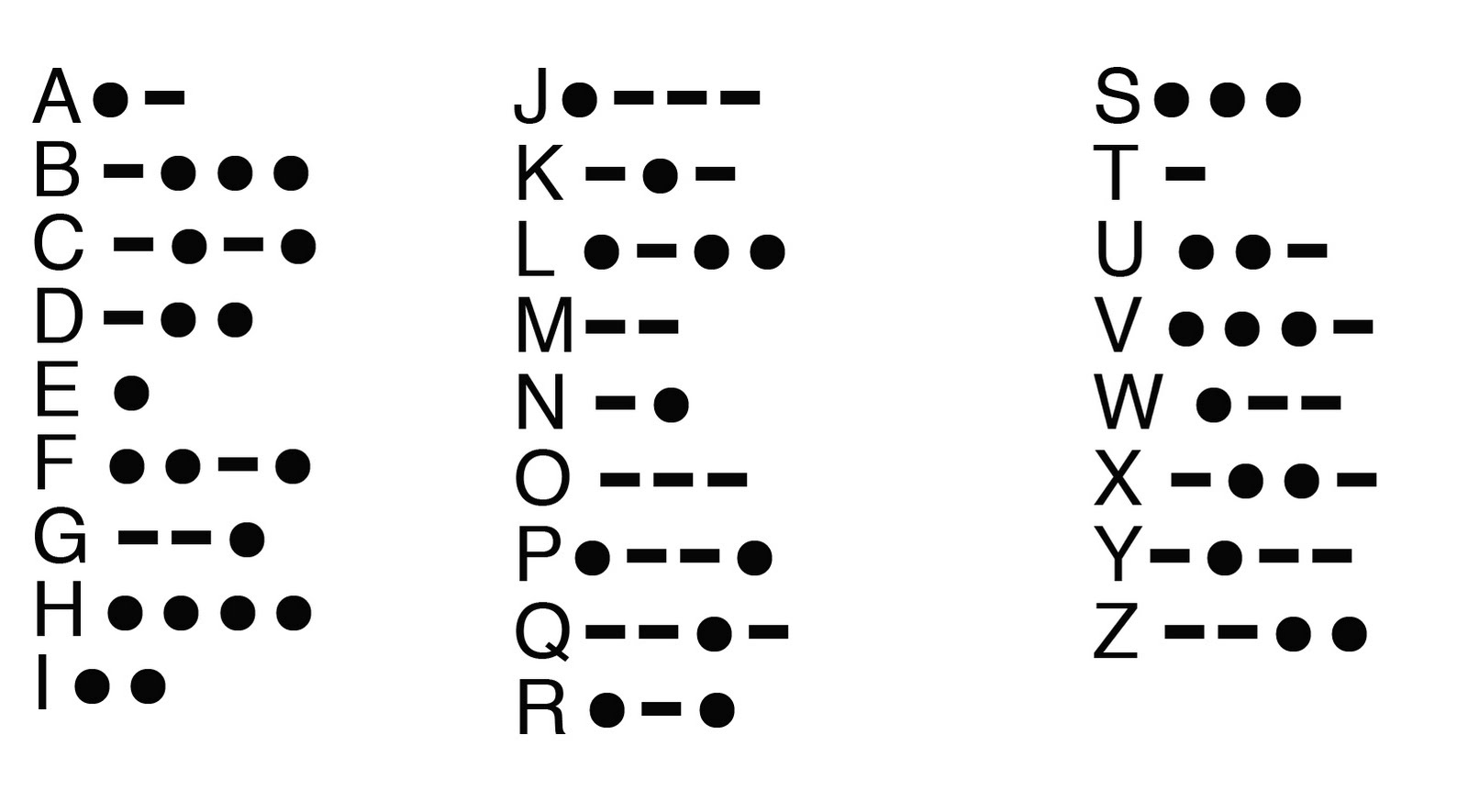
For example in order to send the message "sos help", your program
would flash the LED in the following sequence:
DOT IMD DOT IMD DOT LETTER_PAUSE DASH IMD DASH IMD DASH LETTER_PAUSE DOT IMD DOT IMD DOT
WORD_PAUSE
DOT IMD DOT IMD DOT IMD DOT LETTER_PAUSE DOT LETTER_PAUSE DOT IMD DASH IMD DOT IMD DOT LETTER_PAUSE DOT IMD DASH IMD DASH IMD DOT
The sequence "DOT DOT DOT" is code for the letter "s", "DASH DASH DASH" the
code for "o". Everywhere there is a DOT in this message your LED would be on
for 1 time unit, everywhere there is a DASH the LED would be on for 3 time
units. Between the DOTs and DASHes is an the intra-mark delay (IMD).
During the IMD the LED must be turned off for 1 time unit. Between the
letters of the message, a "LETTER_PAUSE", the LED is turned off for 3 time
units. Between the words of a message, a "WORD_PAUSE", the LED is turned
off for 7 time units. We will standardize a time unit as a fifth of a second
or 200 ms.
Symbol | LED State | Duration | Time
|
DOT | On | 1 time unit | 200ms
|
DASH | On | 3 time unit | 600ms
|
IMD | Off | 1 time unit | 200ms
|
LETTER_PAUSE | Off | 3 time unit | 600ms
|
WORD_PAUSE | Off | 7 time unit | 1400ms
|
Lab 2 assignment
You may work in teams of one or two on this lab. Your first task is
to write a set of functions that you will use to complete the lab.
Write a pair of function, "microSecondDelay" and "milliSecondDelay",
from the inLab to generate delays using NOPs. Make sure that these
functions are properly tuned to accurate delays.
Write a function called "convert" that takes as input a lower-case
ASCII character
and returns a uint8_t that is the index of the letter in the alphabet.
For example, convert('a'); returns 0 while convert('k'); should return 10.
You can write this function in a single line of code if you figure out
how to subtract 'a' from the ASCII value of the input. The convert
function should return 0 for any character value outside 'a' - 'z'.
Write a function called "blink" that takes as input the ASCII code for
a letter and outputs the LED blink pattern for that letter.
Inside of the blink function, define a Morse code letter array
that stores the dot/dash patterns for all 26 letters. For example,
uint8_t morseCode[26][5] = {
{DOT, DASH, END}, // a
{DASH, DOT, DOT, DOT, END}, // b
{DASH, DOT, DASH, DOT, END}, // c
The blink function should call the convert function to convert the ascii
input letter to the blink function into an index into the morseCode array.
You should use #define values for the symbols DOT, DASH and END and others.
For example, the top of my program looks like the following. You will need
to include definitions for INTRA_LETTER_DELAY and INTRA_WORD_DELAY.
#define TIME_UNIT 200
#define DOT_DELAY 1*TIME_UNIT
#define DASH_DELAY 3*TIME_UNIT
#define INTER_LETTER_DELAY 1*TIME_UNIT
#define DOT 0
#define DASH 1
#define END 2
Write a function called "main", yeah the top-level program, that
waits for the upper button to be pressed (and released) and then
transmits the the Morse code blink sequences for the letters of
a message contained in a character array. If the button is pressed
while a character is being blinked out, the button press should be
ignored. After your program has completed transmitted the Morse
code sequence for the message, it should go back and wait for another
button press (and release) and then transmit the Morse code blink
sequence for the letters of a message contained in the character array,
etc....
Program requirements:
- No global variables,
- Use an initialized string for the message in main. For example,
char msg[9] ="sos help"; Your message
should only contain lower case letters and no numbers. The compiler
will null terminate the string for you. In other words, the compiler
will add a '\0' character
to the end of the string for you,
- Write the following functions:
- void microSecondDelay(uint16_t us);
- void milliSecondDelay(uint16_t ms);
- uint8_t convert(char letter);
- void blink(char letter);
Turn-in
You may work with a single partner (or alone) to complete this lab.
Submit your main.c file on Canvas Lab#2 using the instructions posted
there. You should take note of the Rubric that will be used to evaluate
your assignment. Please form a group before submitting using the
instructions posted on Canvas. You will demonstrate your code at the
beginning of lab.