Homework 2 - Creature
This assignment is due by September 6, 2012 by 11:59pm.
Part I - Create a Son of a Creature Man
For this assignment, you will write an OpenGL/GLUT program that displays a 2D animated and interactive creature/character/vehicle/etc. Your creature must be made up of OpenGL primitives, at least one of which is solid, and drawn in a hierarchical fashion. In other words, the eyes and mouth should be drawn with respect to the head and the head with respect to the body. A carefully placed call to glTranslatef() could be used to move entire portions of the creature's body.
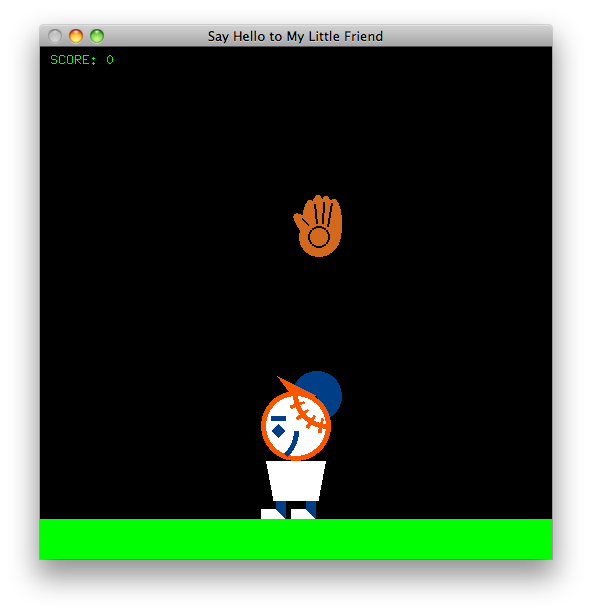 Mr. Met is ready for a day at the park.
The creature must have some form of constant animation regardless of user interaction (head bobbing side to side, arms waving up and down, etc.). The user must be able to move the creature around the screen with the keyboard and the creature must stay on screen so as not to be lost forever. The creature must also interact with the mouse (change the creature's color, expression, fire a laser beam, etc.) and the interaction must be based on the position of the mouse (the creature runs away from the mouse, it fires a homing missile at the mouse, etc). Your program must register a callback for the keyboard, mouse, passive motion, and timer (it is generally not good practice to rely on glutIdleFunc()). Feel free to use the special keyboard keys as well (if you wish to control the creature by the arrow keys).
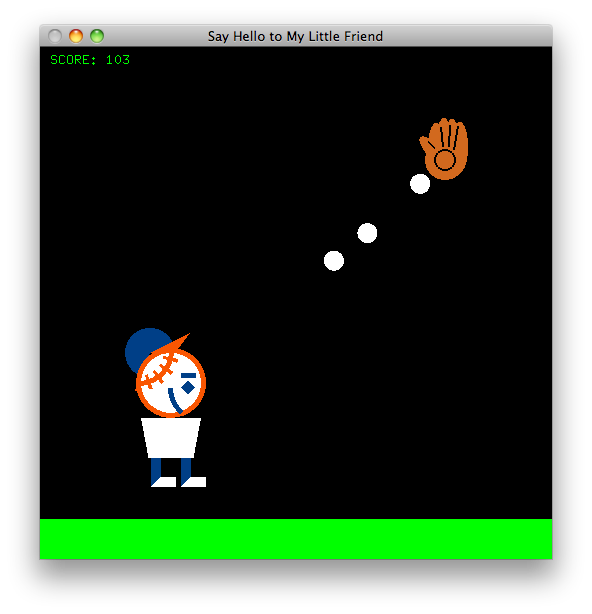 Help Mr. Met warm up for the big game!
Have fun and be creative! Don't be limited to just the suggestions above.
Part II - Website
Update the webpage that you submitted with HW1 to include an entry for this homework assignment. As usual, include a screenshot (or two) and a brief description of the program, intended to showcase what your program does to people who are not familiar with the assignment.
Part III - Questions
Briefly answer the questions below. Include your response in the README.txt file.
- Provide a series of OpenGL calls that will accomplish the following transformations. Your answer should begin and end with glPushMatrix(), glPopMatrix() and there is a function drawMonogram() which takes care of drawing the ND monogram to the screen.
- As seen in class, it is very simple to rotate an object and have it spin in its own object space. The monogram is located at (x, y). How can we rotate the monogram around a fixed point (i, j) without the object spinning but staying stationary around its own local axes? An angle of rotation θr is provided and updated each frame to simulate the animation. (Refer to the example from the end of class on Tuesday, August 28).
- We have discussed the Shear transformation in class. OpenGL does not provide a built in glShearf() as it does for translate, rotate, or scale. Provided an angle of shear θs, how can you tell OpenGL the necessary transformation matrix and apply the matrix to the drawing of the monogram? (Hint: Your answer will not require any reference to glTranslatef(), glRotatef(), or glScalef().)
Documentation
With this and all future assignments, we expect you to appropriately document your code. This includes writing comments in your source code - remember that your comments should explain what a piece of code is supposed to do and why; don't just re-write the code says in plain English. Comments serve the dual purpose of explaining your code to someone unfamiliar with it and assisting in debugging. If you know what a piece of code is supposed to be doing, you can figure out where it's going awry more easily.
Proper documentation also means including a README.txt file with your submission. In your submission folder, always include a file called README.txt that lists:
- Your Name / netID
- Homework Number / Project Title
- A brief, high level description of what the program is / does
- A usage section, explaining how to run the program, which keys perform which actions, etc.
- Instructions on compiling your code
- Notes about bugs, implementation details, etc. if necessary
Grading Rubric
Your submission will be graded according to the following rubric:
20% | Creature is correctly drawn with OpenGL primitives. Draw calls are structured hierarchically, with some form of model instancing [1]. Creature must be broken down into function calls that handle smaller components. |
25% | Creature is in a state of constant animation, even when the user is not interacting with it. |
20% | Creature responds to keyboard presses by moving around the screen. Proper bounds checking is performed to prevent the creature from moving off-screen. Pressing ESC closes the program. |
20% | Creature responds to mouse clicks and mouse movement. The response is based on the location of the mouse. |
5% | Questions from Part III are answered and included in the README.txt file. |
5% | Submission includes source code, Makefile, and README.txt. Source code is well documented. Webpage named <afsid>.html submitted and updated with screenshot from latest assignment. |
5% | Submission compiles and executes in the lab machine environment. |
[1] In other words, a call to drawCreature() would be made up of calls to drawHead() and drawBody(). Within drawHead() are two calls to drawEye() to place each eye on the head. A properly placed call to glTranslatef() could move the entire head with respect to the creature. Each component should be drawn with respect to the component it is attached to (i.e. eye locations are set as offset from center of head) rather than being specified with respect to the center of the creature. Individual components can then be moved hierarchically. If there are any questions, feel free to post a question to Piazza.
Submission
Please update your Makefile so that it produces an executable with the name hw2. When you are completed with the assignment, submit the source code, Makefile, and READEME.txt to the following directory:
/afs/nd.edu/coursefa.12/cse/cse40166.01/dropbox/<afsid>/hw2/
Similarly, title your webpage <afsid>.html (e.g. jpaone.html) and submit it to:
/afs/nd.edu/coursefa.12/cse/cse40166.01/dropbox/<afsid>/www/
Place any screenshots or other images used on the webpage in:
/afs/nd.edu/coursefa.12/cse/cse40166.01/dropbox/<afsid>/www/images/
This assignment is due by September 6, 2012 by 11:59pm.
|